Python Notes and Examples
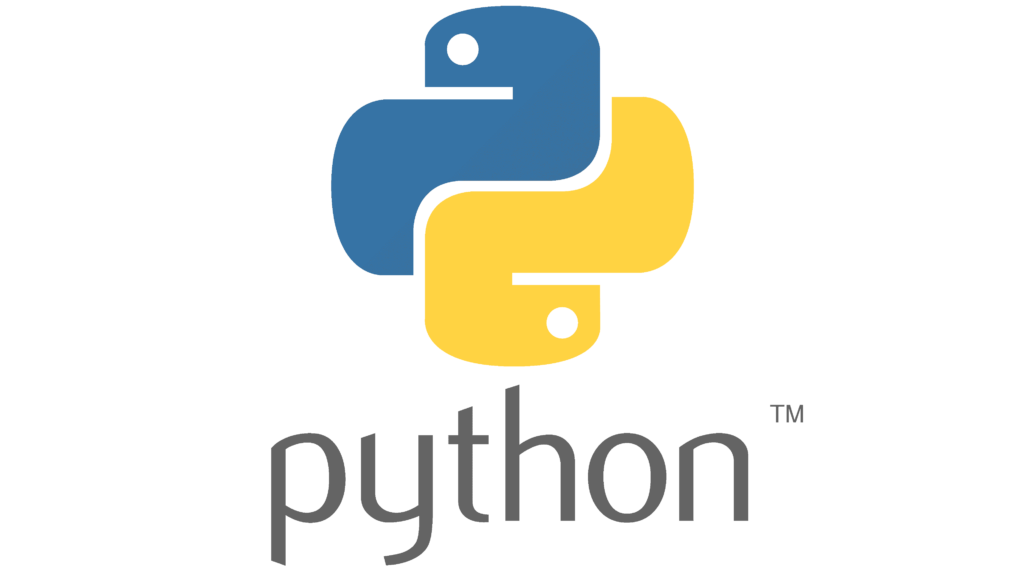
Here are my notes from different courses I’m taking.
Table Of Contents
Courses and resources
https://www.udemy.com/course/learn-python-and-ethical-hacking-from-scratch/
https://tryhackme.com/room/pythonbasics
https://tryhackme.com/room/pythonforcybersecurity
Notes from ‘Learn Python & Ethical Hacking From Scratch‘
Lecture 1 – MAC Address Changer
https://docs.python.org/3/library/subprocess.html
Change MAC address using subprocess
#!/usr/bin/python3
# Import will import a python module. For example here I'm importing subprocess.
# Subprocess is used to run system commands on either linux or windows.
import subprocess
# In pycharm I can use ctrl + d to copy and paste the same line under it.
# Here Im telling python to take down eth0, change its mac, and start it up again
subprocess.call("ifconfig eth0 down", shell=True)
subprocess.call("ifconfig eth0 hw ether 00:11:22:33:44:55", shell=True)
subprocess.call("ifconfig eth0 up", shell=True)
Script upgrade using variables
#!/usr/bin/python3
import subprocess
# Here we are using variables. We are setting interface to eth0 and new_mac to the 00:11...
# We are also printing a statement to the user, and appending the interface and new mac to its easier to understand what is happening.
interface = "eth0"
new_mac = "00:11:22:33:44:77"
print("[+] Changing the MAC address for " + interface + " to " + new_mac)
# We replace the hardcoded interface and mac to using the variables instead.
subprocess.call("ifconfig " + interface + " down", shell=True)
subprocess.call("ifconfig " + interface + " hw ether " + new_mac, shell=True)
subprocess.call("ifconfig " + interface + " up", shell=True)
# We are printing ifconfig eth0 and grepping the mac to show the user that the mac have actually changed
print(subprocess.call("ifconfig " + interface + " |grep ether", shell=True))
Input from user
#!/usr/bin/python3
import subprocess
# Here we are asking a user to input an interface and a new mac. So when executing the script it will look like the pic below.
interface = input("Interface > ")
new_mac = input("new mac > ")
print("[+] Changing the MAC address for " + interface + " to " + new_mac)
subprocess.call("ifconfig " + interface + " down", shell=True)
subprocess.call("ifconfig " + interface + " hw ether " + new_mac, shell=True)
subprocess.call("ifconfig " + interface + " up", shell=True)
print(subprocess.call("ifconfig " + interface + " |grep ether", shell=True))
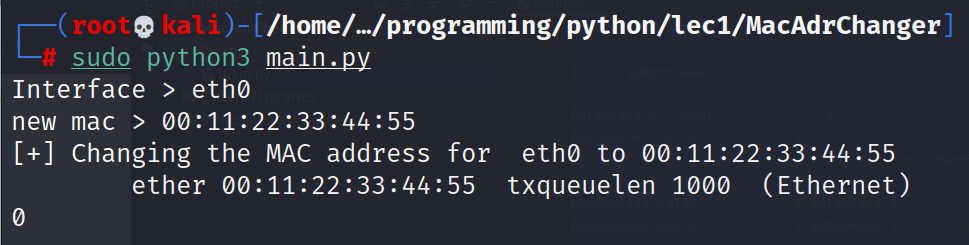
Handling user input
The above example is not a secure way as any user can input whatever they like in the inputfield.
For example
python3 main.py
interface > whoami; id ;
The above command will execute id
command since I’ve added and AND statement.
A more secure way to do it is like this
#!/usr/bin/python3
import subprocess
interface = input("Interface > ")
new_mac = input("new mac > ")
print("[+] Changing the MAC address for " + interface + " to " + new_mac)
# Here we are providing a list of commands. So the variable interface will be treated as an argument for ifconfig, instead of a seperate command as show above.
subprocess.call(["ifconfig", interface, "down"])
subprocess.call(["ifconfig", interface, "hw", "ether", new_mac])
subprocess.call(["ifconfig", interface, "up"])
print(subprocess.call("ifconfig " + interface + " |grep ether", shell=True))
An example of training to hijack the script. As you can see that didnt work because ifconfig
does not have an argument named eth0;id
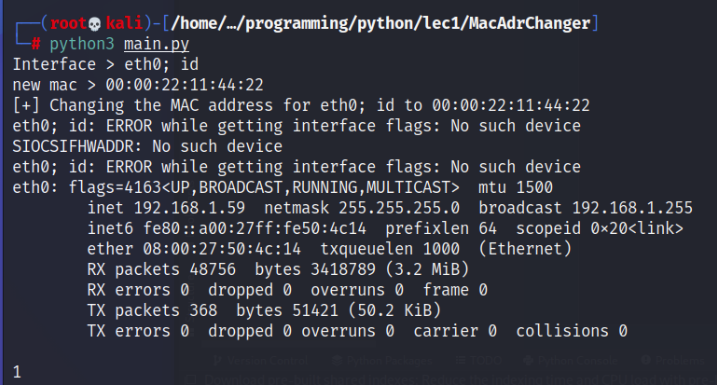
Command-line arguments
#!/usr/bin/python3
import subprocess
import argparse
# Everything that start with a capital letter is a class. Class is a code or blueprint for the object. It determine
# what we can do with the parser object.
parser = argparse.ArgumentParser()
# Here we are saying that when a user specify their argument -i, it will save the output to dest=interface.
# Same with the -m.
# And we are telling it to show a help menu if a user types -h or --help.
parser.add_argument("-i", "--interface", dest="interface", help="Interface to change it's MAC address")
parser.add_argument("-m", "--mac", dest="new_mac", help="New MAC address")
# Then we parse the input. Allow the object to under what the user have typed and handled it.
options = parser.parse_args()
# We are then saving the output from the parser to interface. Options contains the value that the user inputs.
# Then we just type options.interface to get the output from --interface and options.new_mac to get the output from
# --mac.
interface = options.interface
new_mac = options.new_mac
print("[+] Changing the MAC address for " + interface + " to " + new_mac)
subprocess.call(["ifconfig", interface, "down"])
subprocess.call(["ifconfig", interface, "hw", "ether", new_mac])
subprocess.call(["ifconfig", interface, "up"])
print(subprocess.call("ifconfig " + interface
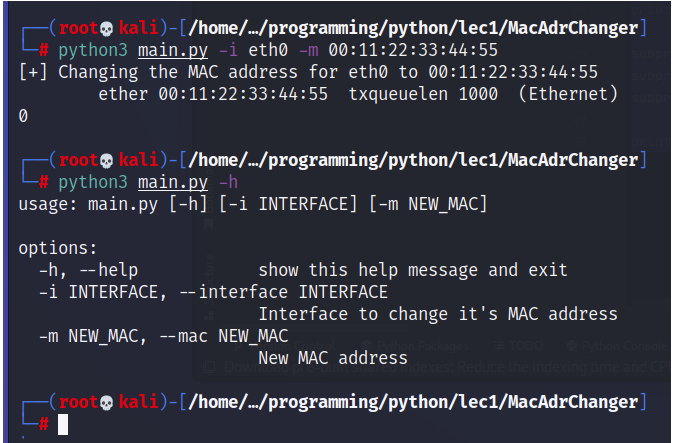
Functions
#!/usr/bin/python3
import subprocess
import argparse
# To define a functions start by typing "def" and a function name. Then specify the input the functions take. In order to change the mac we need an interface and a mac adress, so we will use two variables. Then variable names can be whatever. So if I type X instead of interface, I have to type X in all the following code in the function.
# Then add the code that the function will run by typing the code under it. See the ident.
def change_mac(interface, new_mac):
print("[+] Changing the MAC address for " + interface + " to " + new_mac)
subprocess.call(["ifconfig", interface, "down"])
subprocess.call(["ifconfig", interface, "hw", "ether", new_mac])
subprocess.call(["ifconfig", interface, "up"])
parser = argparse.ArgumentParser()
parser.add_argument("-i", "--interface", dest="interface", help="Interface to change it's MAC address")
parser.add_argument("-m", "--mac", dest="new_mac", help="New MAC address")
options = parser.parse_args()
# If we never call the function, it will not run the code in the function, so we have to call it as shown below.
# In the function we know that we have to give it an interface name and a new mac. So we will use the argparse options.interface and options.new_mac as we saved them in the parser.
change_mac(options.interface, options.new_mac)